Comprehensive Guide to Flutter Android Development
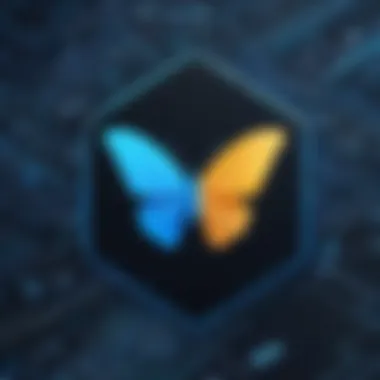
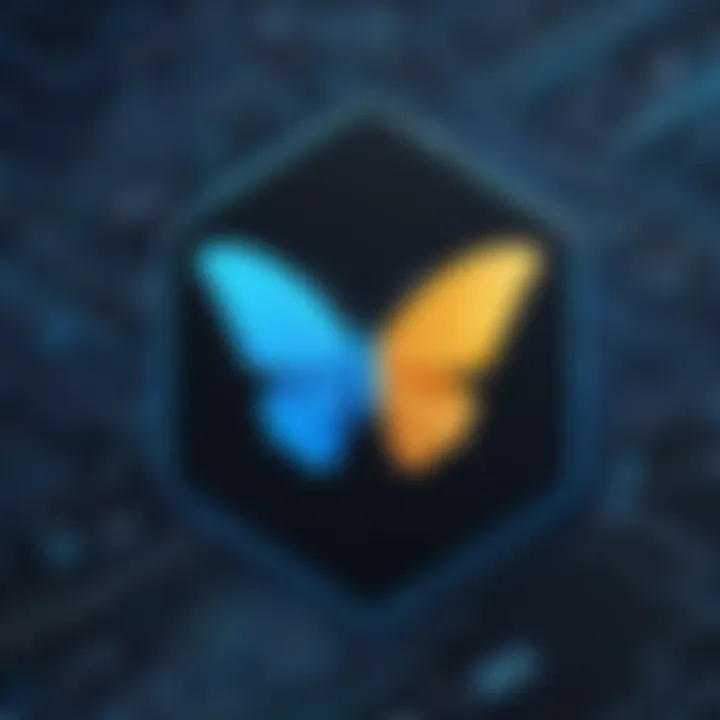
Intro
In the rapidly evolving landscape of mobile application development, Flutter has emerged as a powerful framework that enables developers to create natively compiled applications for mobile, web, and desktop from a single codebase. This guide offers a thorough exploration of Flutter's capabilities, specifically focusing on its application in Android development. We will delve into its architecture, the benefits it offers over traditional frameworks, and the fundamental concepts that underpin its operation. Through a detailed examination of essential tools, performance optimization strategies, and testing methodologies, this guide will equip software developers, IT professionals, and budding students with the knowledge required to harness the full potential of Flutter.
Software Overview
Key Features
Flutter stands out in the crowded field of development frameworks due to several notable features:
- Fast Development: The Hot Reload feature allows developers to see changes in real-time without losing the state of the application.
- Expressive UI: Rich and customizable widgets ease the development of beautiful UIs.
- Native Performance: Flutter compiles to native machine code, ensuring that performance is at par with native applications.
- Cross-Platform: Develop for multiple platforms using a single codebase, reducing time and resources.
System Requirements
To effectively develop applications using Flutter, certain system requirements must be met. Here are the basic requirements:
- Operating System: Windows, macOS, or Linux
- Disk Space: At least 1.5 GB (not including disk space for IDE/tools)
- Tools: An editor such as Visual Studio Code or Android Studio
Additionally, ensure that you have the latest version of the Android SDK installed to facilitate development.
In-Depth Analysis
Performance and Usability
Flutter's architecture contributes to its performance. The framework utilizes a high-performance rendering engine, which enhances the responsiveness of applications. Because Flutter renders UIs directly onto the screen, it bypasses the need for a bridge to interact with the underlying platform, thus reducing latency and increasing fluidity. As a result, applications built with Flutter tend to feel snappier.
Usability is another area where Flutter excels. The framework is designed with developer experience in mind. The clear documentation and active community support ensure that developers can quickly find solutions to common problems.
Best Use Cases
Flutter is remarkably versatile, making it suitable for various use cases in mobile app development:
- Startups: Quick development times favor startups looking to launch Minimum Viable Products (MVPs).
- Prototyping: The ability to iterate and test ideas rapidly aids in the prototyping phase of projects.
- Multi-Platform Applications: Organizations aiming for a consistent user experience across Android and iOS benefit greatly from Flutter’s cross-platform capabilities.
"Flutter simplifies the development process by minimizing code duplication while maintaining native performance." - Flutter Developer Community
Prelude to Flutter
Flutter is an open-source UI software development kit created by Google. It allows developers to build natively compiled applications for mobile, web, and desktop from a single codebase. Flutter’s relevance in today’s software development landscape cannot be overstated; its performance, flexibility, and growing ecosystem present a powerful toolset for building beautiful applications.
Understanding Flutter’s significance starts with grasping its purpose in modern app development. As the demand for cross-platform solutions escalates, Flutter emerges as a compelling choice that not only streamlines development workflows but also ensures high-performance outputs on varied platforms. For developers, this means less time is spent maintaining separate codebases for iOS and Android. The focus then shifts to creativity and user experience rather than grappling with fragmented code.
Moreover, Flutter is noteworthy for its architecture, built around the concept of widgets. Everything in Flutter is a widget, providing a consistent framework across different platforms. Widgets are not merely components; they define the entire look and feel of an application, allowing for high degrees of customization and complex layouts.
Understanding Flutter's Purpose
Flutter serves a crucial role in addressing the complexities involved in mobile application development. It aims to reduce overhead costs associated with maintaining multiple codebases while providing developers with the tools necessary to create rich, interactive user experiences. A significant advantage here is how Flutter allows developers to
- Write once and deploy across multiple platforms.
- Utilize a single programming language, Dart, simplifying the development process.
- Leverage hot reload for rapid iteration, which remarkably accelerates the development cycle.
This focus on efficiency is what makes Flutter appealing, especially for businesses seeking to launch their applications swiftly without sacrificing quality.
Origins and Evolution of Flutter
Flutter's inception dates back to 2015, with its first stable release occurring in December 2018. Initially, Flutter was focused on mobile app development. However, the framework's capabilities have expanded significantly since then. The introduction of Flutter Web brought the possibility of building web applications with the same codebase, increasing its versatility. The development community has embraced Flutter, contributing to its comprehensive array of libraries and plugins, enhancing its functionality.
Today, Flutter is not merely a niche framework but a robust ecosystem. It is backed by substantial community support and regular updates from Google. The evolution of Flutter highlights a commitment to continually adapt to developers' needs while embracing new technologies and practices. This adaptability is essential in a fast-paced industry, making Flutter a relevant tool for future developments.
Flutter represents a shift in how applications are built, focusing on performance and flexibility, appealing to developers and businesses alike.
Architecture of Flutter
The architecture of Flutter is a critical aspect that influences its performance, usability, and overall capabilities in Android development. Understanding this structure provides insights into how Flutter manages to offer rapid development cycles and high-performance applications. This section dissects the various components of Flutter's architecture, detailing how they work together to create seamless experiences for both developers and users.
Core Components of Flutter
Flutter's architecture is built around several fundamental components that play important roles.
- Widgets: Everything in Flutter is a widget, which means even layouts are created with widgets. This uniformity simplifies the development process. Widgets can be stateful or stateless. Stateful widgets maintain state information, while stateless widgets do not.
- Rendering System: Flutter employs a high-performance rendering engine known as Skia. This engine allows Flutter to draw directly to the screen, providing smooth animations and transitions.
- Dart Platform: The underlying programming language for Flutter is Dart. Dart is designed to be easy to learn and enables quick compilation to optimized native code.
- Flutter Framework: This is a collection of libraries that provide APIs to make it easier to build apps. The Flutter framework simplifies processes such as input handling, animations, and gestures.
These core components work synergistically to deliver a robust development environment. By grasping these parts, developers can create applications that are visually appealing and functionally efficient without excessive overhead.
Flutter Engine and its Role
The Flutter engine serves as the backbone of the framework, responsible for crucial tasks that impact application performance and rendering. The engine has several core responsibilities:
- Low-level Rendering: It handles rendering via the Skia engine. This means that developers can achieve complex graphical effects with ease.
- Engine APIs: It exposes APIs needed for tasks such as image loading, text rendering, and input operations. These APIs interact with the underlying platform, whether it be Android or iOS.
- Dart Virtual Machine: The engine also comes equipped with a Dart virtual machine, facilitating hot reloads. This feature allows developers to see updates instantly during the development phase, significantly speeding up workflows.
- Platform Integration: Flutter engine supports native code and enables calling custom platform-specific APIs. This is essential for developers who wish to leverage device-specific features in their applications.
In summary, the Flutter engine is indispensable as it optimizes app performance and enhances developer productivity. This efficiency is one of many reasons why Flutter has gained popularity among developers aiming for high-quality mobile applications.
To effectively harness Flutter’s capabilities, a thorough understanding of its architecture is essential.
Advantages of Using Flutter for Android Development
Understanding the advantages of using Flutter for Android development is fundamental for developers who wish to harness the full potential of this framework. Flutter not only simplifies the development process but also enhances the overall efficiency and performance of the applications developed. This section highlights the critical elements, benefits, and considerations about Flutter that can help guide developers in their decision-making process regarding application development.
Cross-Platform Compatibility
One of the most significant advantages of Flutter is its cross-platform capabilities. This framework allows developers to write a single codebase that operates seamlessly across various platforms, including Android and iOS. This compatibility is driven by the Dart programming language and Flutter's unique rendering engine, which creates a native-like experience on both operating systems.
Developers can save time and resources since they do not need to write separate code for each platform. Reusing code minimizes the chances of inconsistencies between different versions of the app, leading to easier maintenance. Furthermore, Flutter enables quick updates and bug fixes, which are invaluable in today's fast-paced development environment.
Developers increasingly appreciate the ability to extend applications to other platforms such as web and desktop without starting from scratch. This feature opens numerous possibilities for businesses targeting diverse customer bases across multiple devices. Overall, cross-platform compatibility lays a strong foundation for quicker and more efficient application development.
Fast Development Cycle
Flutter significantly accelerates the development cycle, providing a compelling advantage over traditional methods. The "hot reload" feature stands as a testament to this claim. With hot reload, developers can make changes to the code and witness those changes in real time, eliminating the need to restart the application entirely. This not only enhances productivity but also encourages experimentation with various UI designs and features.
Moreover, Flutter's rich set of pre-built widgets allows developers to implement UI designs quickly. Developers can customize these widgets according to their requirements, thus streamlining the design process. The quick turnaround helps teams meet deadlines without compromising the quality of the final product.
In addition to hot reload, Flutter offers robust testing tools directly integrated into the framework, further simplifying the testing process. A faster development cycle ultimately results in quicker delivery of applications to the market and enhances overall business agility.
Rich Widget Catalog
Flutter comes equipped with a rich catalog of widgets that facilitate the creation of aesthetically pleasing and highly functional user interfaces. These widgets are categorized into material design and cupertino, aligning with the look and feel of Android and iOS applications respectively. This extensive selection simplifies the UI development process while ensuring consistency across different screens of the app.
The flexibility offered by these widgets allows developers to design complex interfaces without intensive coding. It allows for easy customization, enabling developers to adapt the appearance and behavior of applications as per user preference. Flutter’s widget system also follows a composition model, meaning that developers can easily combine simple widgets to create more intricate layouts.
For instance, basic widgets like text, buttons, and images can be combined to build more complex structures, saving time and building efficiency. This rich widget catalog enhances developers' creativity and helps them to deliver visually appealing applications that resonate with users.
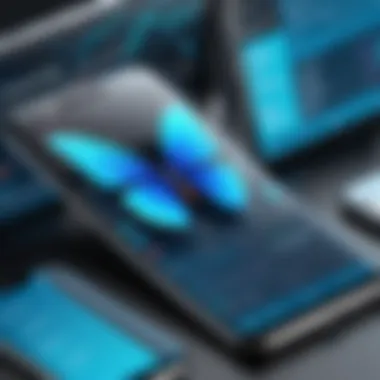
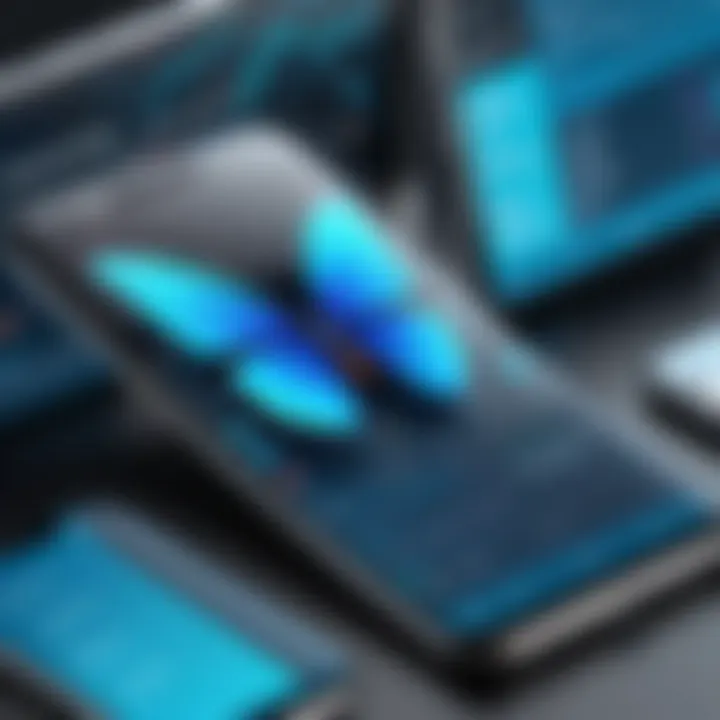
In the ever-evolving landscape of mobile application development, choosing a framework like Flutter can lead to substantial advantages in cross-platform functionality, development speed, and design capabilities.
Foundational Concepts in Flutter Development
Understanding the foundational concepts in Flutter development is essential for any developer who aims to create robust applications. This section will outline key elements of Flutter that contribute to its effectiveness and efficiency as a framework. A solid grasp of these concepts will enable developers to harness Flutter’s full potential.
Dart Language Overview
Dart is the programming language behind Flutter. It plays a critical role in building applications efficiently. Dart is object-oriented and provides features such as strong typing, asynchronous programming, and a rich set of libraries. These features allow developers to write clean and maintainable code.
One of the significant advantages of Dart is its Just-In-Time (JIT) and Ahead-Of-Time (AOT) compilation. With JIT, developers can run and test their apps quickly during development, while AOT provides optimized performance for the production version of the app.
Notably, Dart’s syntax is similar to languages like Java and JavaScript, easing the learning curve for developers familiar with these languages. The language also facilitates lazy loading and hot reload, which significantly enhances the development experience.
"Dart is designed for client-side development, which makes it the perfect match for Flutter's innovative architecture."
Building User Interfaces with Widgets
In Flutter, everything is a widget. This is a fundamental concept that differentiates Flutter from other frameworks. Widgets are the building blocks of the user interface. They can be simple, like Text and Image, or complex, like Layout widgets that handle arrangements of other widgets.
Widgets support a reactive programming model. Whenever a widget's state changes, Flutter rebuilds the widget tree. This approach ensures that the UI remains responsive and reflects the current state of the application. Understanding how to compose and manipulate widgets is crucial for developing efficient applications.
Moreover, Flutter provides a rich catalog of pre-built widgets that follow Material Design guidelines and iOS design principles. Developers can customize these widgets or create their own, offering flexibility in design and functionality.
To illustrate, here is a simple example of a widget tree that creates a basic Flutter application:
In this example, the widget sets up the application. The widget provides structure for the UI, while widgets display a simple message.
Understanding how to utilize and combine widgets is crucial for successful Flutter development. By mastering these foundational concepts in Flutter, developers can create intuitive and engaging applications.
Development Tools and Environment Setup
Setting up the right development tools and environment is crucial for effective Flutter Android development. The quality and structure of your workspace can significantly influence your productivity and the overall success of your project. Ensuring that you have the right tools at your disposal is an essential first step before diving into the actual coding of your application.
Installing Flutter SDK
To begin with, the Flutter Software Development Kit (SDK) must be installed. The Flutter SDK contains all the core libraries and tools needed to build, test, and deploy your Flutter applications. It’s the fundamental block that developers work with when creating apps with Flutter.
Installing the Flutter SDK is a straightforward process. Here’s how to do it step by step:
- Visit the Flutter website: Start by going to www.flutter.dev. This website contains official installation guides and documentation.
- Download the SDK: Select the version suited for your operating system—Windows, macOS, or Linux.
- Extract the download: The SDK comes as a compressed file. Unzip the file to a directory on your machine. For macOS and Linux, it's recommended to place it in the home directory.
- Update your PATH: Add the Flutter bin directory to your system PATH. This lets you run Flutter commands from any command line terminal.
- Run the Flutter Doctor: After installation, run . This command checks your environment and displays a report of the status of your Flutter installation. It will also prompt you for missing dependencies, like Xcode or Android Studio.
In summary, installing the Flutter SDK is a fundamental step in setting up your development environment. It ensures that you have access to all necessary tools and libraries required for Flutter development, streamlining your workflow.
Choosing an Integrated Development Environment (IDE)
The next critical component is selecting an Integrated Development Environment (IDE). The IDE is where most of the programming work is done, impacting both the efficiency and quality of development. For Flutter, you have several options, the most popular ones being Android Studio and Visual Studio Code.
When choosing an IDE, there are several factors to consider:
- Compatibility: Ensure that the IDE supports Flutter and Dart to take advantage of its features fully. Android Studio comes with built-in support for Flutter, making it a preferred choice for many developers.
- Plugins and Extensions: IDEs like Visual Studio Code offer a robust marketplace for extensions. You can find useful plugins to aid in your development process, such as Flutter and Dart plugins which provide syntax highlighting, error checking, and code formatting.
- User Interface: A user-friendly interface is important. It should allow for easy navigation and access to necessary tools and files without clutter.
- Performance: Choose an IDE that performs efficiently on your machine. An IDE that is slow or unresponsive can hinder productivity.
Ultimately, your choice of IDE should match your specific needs and preferences. Both Android Studio and Visual Studio Code have their strengths. Android Studio is rich in features for Android development, while Visual Studio Code is lightweight and supports a wide range of other languages.
"The choice of IDE can significantly impact your development experience and efficiency. Choosing the right one is a step towards smoother and more effective development."
Creating Your First Flutter Application
Creating your first Flutter application is a fundamental step in understanding the Flutter ecosystem. This section is essential as it establishes a baseline for developers regarding how to turn concepts into functioning applications. Flutter's unique features, such as its widget-based architecture and hot reload capabilities, streamline this process, allowing developers to see changes instantly. This not only enhances productivity but also minimizes the learning curve associated with traditional mobile development tools.
By focusing on the initial setup and structure of a Flutter project, developers can grasp how to navigate their development environment effectively. Understanding the project setup is crucial because it fundamentally influences the subsequent app design and function. Additionally, properly configuring the environment ensures that developers avoid common pitfalls that may arise later in the development cycle.
Setting Up Your Project
To set up your Flutter project, you need to follow a straightforward sequence of steps. First, ensure that you have installed the Flutter SDK correctly. After installation, you can create a new project using either command line tools or your chosen Integrated Development Environment (IDE).
- Command Line:This command creates a directory called , which houses the basic structure of your Flutter project.
- Setting the Directory:
- Launching the App:This command compiles the application and launches it in your default browser or emulator.
- Open your terminal.
- Run the command:
- Navigate to your project directory:
- Use the command to open the app:
Setting up your project correctly is essential, as it creates a clean slate for your development work. It avoids issues related to misconfiguration that could lead to wasted time later in the process.
Understanding the Main() Function
In any Flutter application, the function serves as the entry point. It is a simple yet crucial method, as it initializes the application and runs the top-level widget. Essentially, this function acts like a command center, instructing the Flutter engine on which widget to load first.
Here, you would typically find a structure similar to this:
This code snippet demonstrates a simple Flutter app that displays "Hello Flutter" in an app bar. The function is particularly important as it takes a widget and makes it the root of the widget tree.
Understanding how the function works is essential for fine-tuning Flutter applications. It lays the groundwork for any further functionality you wish to implement, such as state management, routing, and more.
State Management in Flutter
State management is a crucial aspect of Flutter development. It refers to how data flows through the application and how changes in data affect the user interface. An effective state management strategy enables developers to create applications that are scalable, maintainable, and easy to understand. Flutter has a rich set of tools and techniques for managing state, which can significantly enhance the user experience and developer efficiency.
Poorly managed state can lead to complicated and convoluted code, which hampers maintainability. Understanding how to implement state management correctly can prevent unnecessary re-renders and improve performance.
Understanding State Management
In Flutter, state can be categorized into two types: stateless and stateful. Stateless widgets do not maintain state, while stateful widgets can hold information that can change over the lifetime of the widget. State management in Flutter typically involves managing the state of stateful widgets.
Developers must decide when to rebuild the UI in reaction to state changes. Flutter provides various options to handle this effectively. Some state management methods focus on simplicity, while others favor a more structured approach, meeting different needs based on application complexity.
Popular State Management Solutions
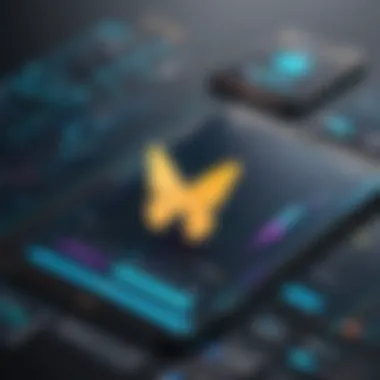
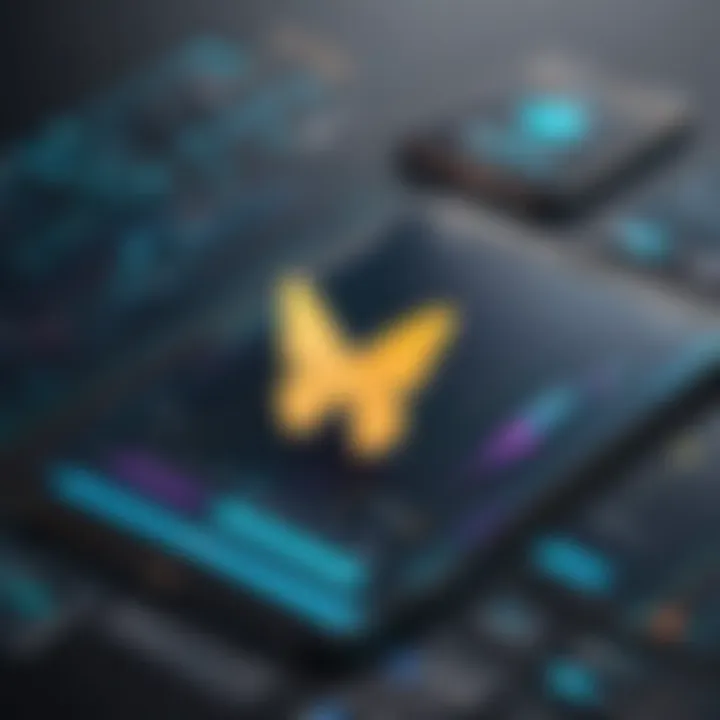
There are several state management solutions available in Flutter, each with its unique characteristics and benefits. Here are three popular options:
Provider
Provider is a widely used state management solution in Flutter. It is known for its simplicity and integration with Flutter's widget tree. One of its key characteristics is the ease of use when it comes to dependency injection.
Advantages:
- Easy to understand: Provider follows a straightforward model, making it accessible, especially for beginners.
- Efficient: It only rebuilds the necessary parts of the widget tree, optimizing performance.
Unique Features:
- Provider uses ChangeNotifier and Consumer widgets for listening to changes, which allows selective rebuilding, making it efficient.
Disadvantages:
- Provider may not be as powerful as some other state management solutions in larger applications, where maintaining complexity becomes a challenge.
BLoC
BLoC, or Business Logic Component, is another popular state management solution. It separates business logic from the user interface entirely. This promotes better testing and reusability of code. The key characteristic of BLoC is its reactive programming model, where data flows through streams.
Advantages:
- Separation of concerns: BLoC keeps UI code clean and focused on presentation rather than business logic.
- Highly testable: It is easier to write unit tests for business logic when separate from UI code.
Unique Features:
- It leverages Dart's Stream and Sinks to manage state, resulting in a highly reactive architecture.
Disadvantages:
- Students new to reactive programming may find BLoC steeper when it comes to learning curves, compared to simpler options.
Riverpod
Riverpod is a more recent addition to the Flutter state management solutions. One of its significant aspects is its compile-time safety, which adds a layer of assurance against runtime errors. It aims to improve on Provider by removing its limitations.
Advantages:
- Scalability: Riverpod scales well for small and large applications, and it does not depend on the widget tree.
- Safe: Compile-time safety helps catch errors early, making it robust for larger applications.
Unique Features:
- It uses providers as a way to declare and expose dependencies throughout the application.
Disadvantages:
- Riverpod can be somewhat complex for beginners to grasp at first, especially if they come from a simple state management background.
Understanding state management is essential for creating efficient and responsive applications in Flutter. Each solution offers unique benefits, and the right choice depends on the specific needs and complexity of the project.
In summary, choosing the right state management solution in Flutter is a foundational step for developers. Whether opting for the straightforward nature of Provider, the robust separation offered by BLoC, or the modern approach of Riverpod, understanding state management options is crucial for delivering excellent Flutter applications.
Routing and Navigation
Routing and navigation are critical elements within any application, especially in mobile app development. In Flutter, effective routing and navigation control user experience and enhance application usability. By structuring the way users move through an app, developers can greatly influence how intuitively it's navigated. This section delves into the architecture that facilitates routing in Flutter, and the differences between named routes and anonymous routes.
Understanding the Navigation Architecture
Flutter’s navigation architecture is built around the concept of a stack of pages or routes. Each page represents a screen in the app. When you navigate to a new page, Flutter adds it to the stack. Whenever the user goes back to a previous page, it removes the top page from the stack. This standard model simplifies navigation tasks, making the user experience straightforward and coherent.
Key Components of Navigation Architecture:
- Navigator: The Navigator widget manages the stack of routes. It provides methods to push or pop routes.
- Routes: Each route is an abstraction of a screen. Instances of routes can be created by defining a .
- Context: The build context is essential in navigating; it provides access to the Navigator instance.
Understanding this structure allows developers to handle transitions between screens effectively. Choosing how to implement routing can make a significant difference in app performance and user satisfaction.
Named Routes vs. Anonymous Routes
In Flutter, you can navigate between pages using either named routes or anonymous routes. Each method has its distinct advantages and best use cases.
- Named Routes: These routes are defined in a centralized manner. They are registered in the main application code. This structure allows for better organization and easy navigation throughout the app. It looks like this:
Apps use named routes primarily for navigation based on consistent paths, enhancing the readability of code and making it easier to manage.
- Anonymous Routes: Anonymous routes do not require naming; developers define them right at the point of navigation. They are often simpler and quicker to implement for transient screens where no direct navigation path is needed.
Using anonymous routes can lead to a more straightforward implementation but could hinder clarity if an application grows significantly. Each method should be considered based on the app's needs.
Important Note: Selecting the right routing method is essential for scalability. Excessive use of anonymous routes can lead to code that is hard to maintain.
Overall, mastering routing and navigation in Flutter enables you're a seamless and engaging user experience. This knowledge becomes crucial as applications grow in complexity and feature sets.
Handling Data in Flutter Apps
Handling data effectively is crucial in Flutter app development. Data forms the backbone of applications, influencing their performance and user experience. Developers need a solid understanding of how to manage data in Flutter to create efficient, responsive, and user-friendly applications. This section covers two primary topics: using APIs to fetch real-time data and local database options to store persistent information.
Using APIs in Flutter
APIs are essential for enabling communication between the app and external services. They allow applications to retrieve or manipulate data hosted on remote servers. In Flutter, making HTTP requests is straightforward, thanks to the http library. This library allows developers to perform GET, POST, PUT, and DELETE operations seamlessly.
Using APIs has several benefits. First, it allows apps to remain lightweight. Instead of storing large amounts of data locally, an app can fetch only what it needs when required. Second, utilizing APIs ensures that users access the most current data, which is especially crucial for applications like news readers or social media. To implement API requests in Flutter, you can follow this basic example:
Being aware of error handling while dealing with APIs is also important. Network issues or invalid responses can lead to application crashes or poor user experience if not handled correctly.
Local Database Options
For many applications, storing data locally becomes a necessity. Flutter offers several options for local data storage. Two of the most common methods are SQLite and Shared Preferences.
SQLite
SQLite is a lightweight, serverless database that is popular among mobile app developers. It offers many advantages for handling structured data efficiently. One of its key characteristics is the ability to perform complex queries using SQL syntax. This allows for more flexibility compared to other storage solutions.
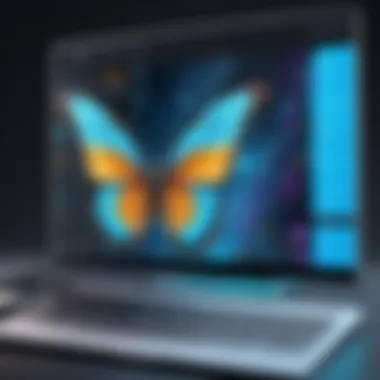
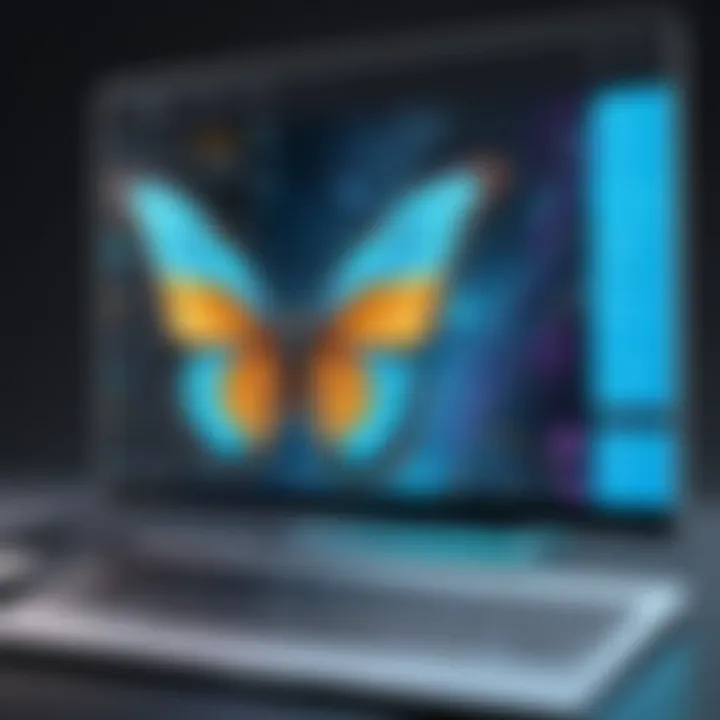
The unique feature of SQLite is its full-text search capabilities, enabling developers to implement robust searching functionaility within their app. This makes it suitable for large datasets where quick search results are required.
However, there are some disadvantages to consider. SQLite databases can increase the app's size due to additional libraries, and managing database migrations can become complex as the application evolves. Despite these considerations, its popularity stems from its efficiency and reliability in data handling for Flutter applications.
Shared Preferences
Shared Preferences is a key-value storage system that is simple to use and offers an excellent way to store user settings and app state. This method is ideal for storing small amounts of data like user preferences or simple configurations.
A notable characteristic of Shared Preferences is its simplicity. It does not require any complex setup, making it quick for developers to implement. For example, saving user preferences can be done quickly with the following code:
The advantage of using Shared Preferences is that it is lightweight, and the performance is excellent for small datasets. However, it is not suitable for more significant data or complex queries, which limits its use in larger applications. Understanding when to use Shared Preferences versus SQLite is vital for effective data management in Flutter apps.
"Choosing the right data management solution can significantly impact app performance and user satisfaction."
In summary, handling data in Flutter apps involves choosing appropriate methods based on use cases, application size, and complexity. Using APIs allows for real-time data access, while SQLite and Shared Preferences offer efficient ways to manage local data.
Testing Your Flutter Application
Testing is an integral component of effective Flutter application development. It ensures that applications function as intended, leading to higher quality software that meets user expectations. This section delves into the importance of testing within the Flutter framework, discussing the distinct aspects of unit testing and integration testing strategies, both vital for maintaining robust applications.
Testing not only helps in identifying issues but also facilitates a better understanding of the code's performance. When testing is effectively implemented, developers can catch bugs early in the development cycle, therefore saving time and resources over the long term. The immediate benefit encompasses enhanced application reliability and ultimately, user satisfaction.
It is essential to adopt a comprehensive testing strategy that balances unit and integration tests. Each type of testing serves unique purposes, strengths, and weaknesses. This balance is key to an effective development workflow.
Unit Testing in Flutter
Unit testing is a fundamental practice in software development. It involves testing individual components or functions of an application in isolation. In Flutter, unit tests are easy to write and execute. This type of testing ensures that each function behaves as expected, reinforcing confidence in the application’s integrity.
A unit test typically includes:
- Setup: Preparing the environment before the test.
- Execution: Running the function or component under test.
- Verification: Checking if the outcome is as anticipated.
The Flutter framework provides a rich set of testing tools that simplify writing unit tests. The built-in package allows developers to create tests and control their execution. Here is a simple example of a unit test in Flutter:
In the above example, the function is tested to ensure it returns the correct sum. This modular testing approach allows for isolated troubleshooting, which is critical for diagnosing issues efficiently.
Integration Testing Strategies
Integration testing extends beyond individual components to examine how various parts of the application interact with each other. In Flutter, integration tests focus on the interaction between multiple components, verifying that they work together smoothly when assembled. This testing phase helps catch unexpected problems resulting from component interactions.
Common strategies for integration testing in Flutter include:
- Widgets Tests: Testing specific widgets and their behavior within the UI context.
- Driver Tests: Automation tools that simulate user interactions, testing UI components in a real device or simulator environment.
- Golden Tests: Comparing UI outputs against stored reference images to ensure consistent rendering across versions.
To implement integration tests in Flutter, the package can be utilized. This package provides functions to write comprehensive integration tests with a clear focus on user scenarios. Here’s a basic setup for an integration test:
Integration tests foster thorough testing coverage of user flows, enhancing the durability of applications made with Flutter. They serve to ensure that all interacting components behave correctly when combined, significantly improving the overall user experience.
In summary, establishing a detailed testing regimen that encompasses both unit and integration testing is essential for the development of reliable and efficient Flutter applications. Regular testing guarantees stability and usability, which are valuable in an increasingly competitive landscape.
Performance Optimization Techniques
In the realm of Flutter Android development, performance optimization plays a vital role in delivering smooth and responsive applications. It is essential for enhancing user experience and retaining user engagement. A well-optimized Flutter application not only runs faster but also consumes fewer resources. This is particularly important on mobile devices, where performance can directly influence battery life and data usage.
Key factors to consider in performance optimization include the efficient use of memory, minimizing widget rebuilds, and asynchronous programming practices. These elements combine to create an application that operates at peak efficiency while maintaining user satisfaction.
Understanding Flutter Performance Metrics
To optimize performance effectively, developers must understand various performance metrics that gauge the application's efficiency. Key performance metrics include:
- Frame Rate: The number of frames rendered per second (FPS). Maintaining 60 FPS is ideal for a smooth user interface.
- CPU Usage: Tracking CPU load can help identify performance bottlenecks in the application.
- Memory Usage: Monitoring memory consumption is critical, as excessive usage may lead to app crashes or slowdowns.
- GPU Rendering: Understanding how graphics are rendered can help in optimizing visual performance.
Developers can monitor these metrics using Flutter's built-in DevTools. Accessing the performance overlay will provide real-time data and assist in pinpointing areas needing improvement.
Effective Use of Widgets
Widgets are the cornerstone of Flutter development. Properly managing widgets can make a substantial difference in performance. Here are some strategies for effective widget use:
- Use Stateless Widgets: Prefer stateless widgets for parts of the UI that do not need to change. They are generally faster and consume less memory.
- Avoid Unnecessary Rebuilds: Minimize widget rebuilding by using keys and maintaining state as needed. This can be achieved through state management solutions like Provider or BLoC.
- Lazy Loading: Implement lazy loading for lists or complex layouts. This will ensure that only visible items are rendered, significantly improving rendering times.
- Optimize Images: Use appropriate image formats and sizes. Flutter has effective tools for image loading, like the package, which can help in managing network resources and improving load times.
In Flutter, understanding how widgets work and optimizing their use is fundamental to building high-performance applications.
By focusing on these aspects, developers can significantly enhance Flutter application performance, leading to a better experience for users and long-term success in app deployment.
Future Trends in Flutter Development
The field of Flutter development is rapidly evolving. Understanding future trends is crucial for developers looking to stay ahead in a competitive landscape. This section highlights key trends, their significance, and potential benefits. It provides insights that can enhance mobile application development in numerous ways.
Emergence of New Libraries and Packages
The Flutter ecosystem is expanding continually, with new libraries and packages emerging to meet diverse developmental needs. This growth allows developers to leverage pre-built components, enhancing the speed and efficiency of project delivery. Libraries such as Dio for API calls and Provider for state management have gained popularity. They simplify coding tasks and improve maintainability.
Additionally, the introduction of packages tailored for specific functionalities, such as animations or Firebase integration, accelerates the development process. It reduces redundancy since developers can utilize existing solutions rather than reinventing the wheel. Keeping an eye on trending packages can provide a competitive edge. Developers can use curated lists from platforms like pub.dev, which ranks packages by popularity and usability.
Flutter Community and Ecosystem Growth
The growth of the Flutter community is notable. As more developers adopt Flutter for mobile development, the community becomes a rich resource for knowledge sharing and collaboration. This growth fosters an environment for innovation. New ideas, solutions, and tools emerge frequently.
Community-driven platforms, such as forums on Reddit, provide a space for developers to discuss patterns, best practices, and troubleshooting techniques. Engaging with the community can lead to discovering trends and tools before they become mainstream. Moreover, participation in community events—like meetups and conferences—can enhance professional networks and open pathways for collaboration.
Epilogue
The conclusion of this article serves as a final reflection on the various aspects of Flutter Android development discussed throughout the guide. This section is crucial as it underscores the primary elements explored, emphasizing their relevance in today's rapidly evolving tech landscape. Flutter offers a robust framework for mobile development, ensuring efficiency and flexibility for developers.
Summarizing Key Takeaways
In summarizing the key takeaways from the guide, several points stand out:
- Versatility: Flutter provides a unique cross-platform experience, allowing developers to create applications for both Android and iOS with a single codebase.
- Rapid Development: The hot reload feature significantly accelerates the development cycle, enabling developers to see changes in real-time.
- Rich Widget Ecosystem: With a comprehensive catalog of customizable widgets, developers can build feature-rich applications that meet various design requirements.
- Community Support: The growing community and resources available continue to enhance Flutter's capabilities and foster best practices in development.
- Performance Optimization: Understanding the performance metrics and utilizing efficient widget strategies can dramatically improve app responsiveness and user experience.
These takeaways provide a solid foundation for developers seeking to leverage Flutter's capabilities effectively in their projects.
Encouraging Continued Learning
Continued learning is essential for every developer, especially in a field as dynamic as mobile app development. As Flutter evolves, staying updated with new features, best practices, and community trends becomes paramount. Here are several ways to engage in ongoing education:
- Online Resources: Websites like en.wikipedia.org and reddit.com offer forums for discussion and resources for learning. Engaging with these platforms keeps one informed.
- Documentation: Always refer to the official Flutter documentation for the latest updates and practices.
- Courses and Workshops: Consider enrolling in online courses or attending workshops focused on Flutter to deepen understanding and gain hands-on experience.
- Networking: Participate in developer meetups or online communities. Sharing knowledge and experiences fosters growth and can lead to collaborative opportunities.
By committing to continuous learning, developers can ensure that they remain competitive and proficient in the ever-changing landscape of Flutter and mobile development.